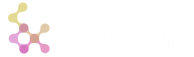
Adverbs
Adverbs are high order constructions that modify the way a verb is applied to data.
Each monad
Applies monadic in the left argument to each element of the right structure.
o){x+1}'4 5 6
5 6 7
o)
Each dyad
Applies dyad to each pair of left and right arguments' elements or pair with one item.
o)1 2 3 +' 4 5 6
5 7 9
o)10+'1 2 3
11 12 13
o)
Each prior
Applies dyad to subsequent pairs of right argument structure.
o)+':1 2 3
1 3 5
o)
... beware of edge cases though:
o){y}':,1
,0N
o){y}':1 2
0N 1
o)
In lambdas, implicit an argument x - current item, y - prior item.
Each prior with initial value
Same as above, but the left argument defines initial value.
o)10*':1 2 2
10 2 4
o)
Each right
Applies dyad to each pair of the left argument and right argument elements.
o)10 100+/:1 2 3
11 101
12 102
13 103
o)
Each left
Applies dyad to each pair of the left argument elements and right argument.
o)10 100+\:1 2 3
11 12 13
101 102 103
o)
Over aka fold
Reduces right argument structure using left dyad. Initial value is different for some verbs.
o)+/1 2 3
6
o){0N!x,y; x+y}/10 3 2 1
10 3
13 2
15 1
16
o)
Boolean vectors behave as vectors of long for some verbs:
o)+/101b
2
o)
o)+/0#0
0
o)
However:
o)*/0#0
1
o):/0#0
0N
o)
Over with initial value
Folds right argument structure using left dyad. Initial value is given explicitly:
o)10*/1 2 2
40
o)
Over "converge"/"fixedpoint"
Repeatedly applies monadic function the left argument until result stops changing:
o){x%2}/100
0
o)
over
with a lambda is applied to a vector, and there is no implicit argument y
in the lambda, then the entire vector is taken as one argument. o) // !!! infinite loop !!!
o) {0N!x; x+1}/1 2 3
2 3 4
3 4 5
...
In this line, x will change infinitely. o){0N!x; (1+x*3)%4}/2 5 3 1
2 5 3 1
1 4 2 1
1 3 1 1
1 2 1 1
1 1 1 1
1 1 1 1
o)
Scan
Acts pretty much the same as "over", but rеturns a structure of the same size.
o)+\1 2 3
1 3 6
o)+\011b
0 1 2
o)
Scan with initial value
"Scan" but with explicitly given initial value.
o)10*\1 2 2
10 20 40
o)
Scan "converge"/"fixedpoint"
Repeatedly applies monadic function in the left argument to the right one, until result stops changing and accumulates all intermediate results:
o){x%2}\100
100 50 25 12 6 3 1 0
o)
scan
with a lambda is applied to a vector, and there is no implicit argument y
in the lambda, then the entire vector is taken as one argument. o) // !!! infinite loop !!!
o) {x+1}\1 2 3
2 3 4
3 4 5
...
In this line, x will change infinitely. o){(1+x*3)%4}\2 5 3 1
2 5 3 1
1 4 2 1
1 3 1 1
1 2 1 1
1 1 1 1
1 1 1 1
o)
"For" loop
Applies monadic verb to the right argument left argument times.
o)5{x+1}/10
15
o)
"While" loop
Applies monadic verb to the right argument while the left argument rеturns true value.
o){x<100}{x*2}/1
128
o)
Scan "For" loop
Applies monadic verb to the right argument left argument times.
o)5{x+1}\10
10 11 12 13 14 15
o)
Scan "While" loop
Applies monadic verb to the right argument while the left argument rеturns true value.
o){x<100}{x*2}\1
1 2 4 8 16 32 64 128
o)
Commute
~
transforms dyadic to have its left and right argument swapped.
Amend/dmend also support commute dyads:
o)a:!5
0 1 2 3 4
o).[a;();~[_];-2]
0 1 2
o)